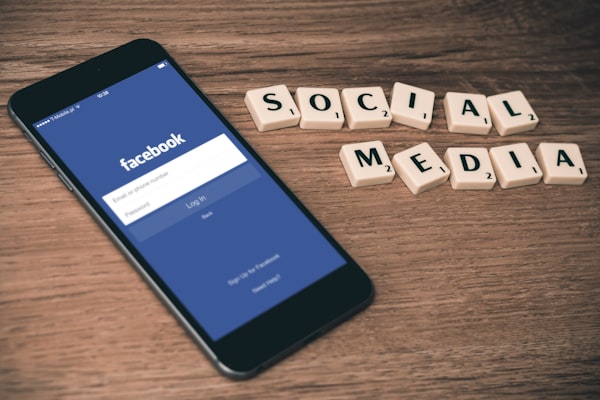
Spring Boot集成 MongDB
pom依赖
<!-- MongoDB -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
yaml文件配置
spring:
data:
mongodb:
# uri格式为mongodb://username:password@host1:port1,host2:port2,...,hostN:portN/database?options
uri: mongodb://root:123456@192.168.0.99:27017/demo01?authSource=admin
#uri 等同于下面的配置
#database: test
#host: 192.168.0.99
#port: 27017
#username: root
#password: 123456
#authentication-database: admin
# 无密码连接方式
mongodb://127.0.0.1:27017
# 配置集群ip:port可以写多个,用逗号隔开
mongodb://用户名:密码@ip:port,ip:port,ip:port/dbname?authSource=admin
实体类
实体类相关注解
@Document:修饰类,用来指定操作集合的名称
@Id:修饰成员变量,用来映射文档的_id的值
@Field:修饰成员便令,用来解决实体类名称与数据库中的key不一致的问题
/**
* 这个实体类仅为举例使用,演示相关注解的使用方法
*/
@Data
@Document(collection = "person")
@AllArgsConstructor
@NoArgsConstructor
public class Person implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private String id;
@Field("username")
private String name;
private Integer age;
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8")
@Field("create_time")
private Date createTime;
}
注入模板类
@Resource
private MongoTemplate mongoTemplate;
插入文档
// 插入单个
Person person = new Person(null, "张三", 18, new Date();
mongoTemplate.insert(person);
// 批量插入
List<Person> list = Arrays.asList(
new Person(null, "张三", 18, new Date()),
new Person(null, "李四", 19, new Date()),
new Person(null, "王五", 20, new Date())
);
mongoTemplate.insert(list);
存储文档
// save方法为更新或插入,根据_id判断,id存在则更新,否则插入
// 需要注意更新是覆盖更新,也就是对象中的null值会覆盖数据库中的数据
mongoTemplate.save(person);
条件对象
查找,更新,删除都可能会使用到query条件对象,下面演示条件如何构建
#################################### 创建Criteria构建条件 ####################################
# 比较语句格式 : Criteria.where("数据库key").xxx
# .is 等于,gt大于,gte大于等于,in包含,it小于,ite小于等于,nin不包含
Criteria criteria = Criteria.where("age").is(18);
# 模糊匹配 写法1
Pattern pattern = Pattern.compile("^.*" + param + ".*$", Pattern.CASE_INSENSITIVE);
Criteria.where("name").regex(pattern);
# 模糊匹配 写法2,举例匹配字符串 三
Criteria.where("name").regex("三");
# AND
Criteria criteria1 = new Criteria().andOperator(criteria1,criteria2,);
# OR
Criteria criteria1 = new Criteria().orOperator(criteria);
Query query = new Query(criteria);
#################################### 使用JSON字符串构造条件 ####################################
String json = "{name:'张三'}";
Query query = new BasicQuery(json);
更新文档
// 条件对象
String id = "6641d28aa7331f57b9e80ff0";
Query query = new Query(Criteria.where("_id").is(id));
// 更新对象
Update update = new Update();
update.set("age",18);
// 更新满足条件的第一条数据
mongoTemplate.updateFirst(query,update,Person.class);
// 更新满足条件的所有数据
mongoTemplate.updateMulti(query,update,Person.class);
删除文档
// 条件对象
String id = "6641d28aa7331f57b9e80ff0";
Query query = new Query(Criteria.where("_id").is(id));
// 删除满足条件的数据,并返回删除条数
DeleteResult remove = mongoTemplate.remove(query, Person.class);
System.out.println("删除条数:" + remove.getDeletedCount());
// 删除满足条件的单个文档,并返回删除的文档
Person result = mongoTemplate.findAndRemove(query, Person.class);
System.out.println("删除的文档数据:" + result.toString());
查询文档
// 查询全部文档
List<Person> all = mongoTemplate.findAll(Person.class);
// byId
Person byId = mongoTemplate.findById("6641d28aa7331f57b9e80ff0", Person.class);
// 条件查询
Query query = new Query(Criteria.where("_id").is("6641d28aa7331f57b9e80ff0"));
List<Person> people = mongoTemplate.find(query, Person.class);
// 条件查询返回第一条数据
Person person = mongoTemplate.findOne(query, Person.class);
// 查找结果排序
query.with(Sort.by(Sort.Order.desc("createTime")));
List<Person> people = mongoTemplate.find(query, Person.class);
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 七十七
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果